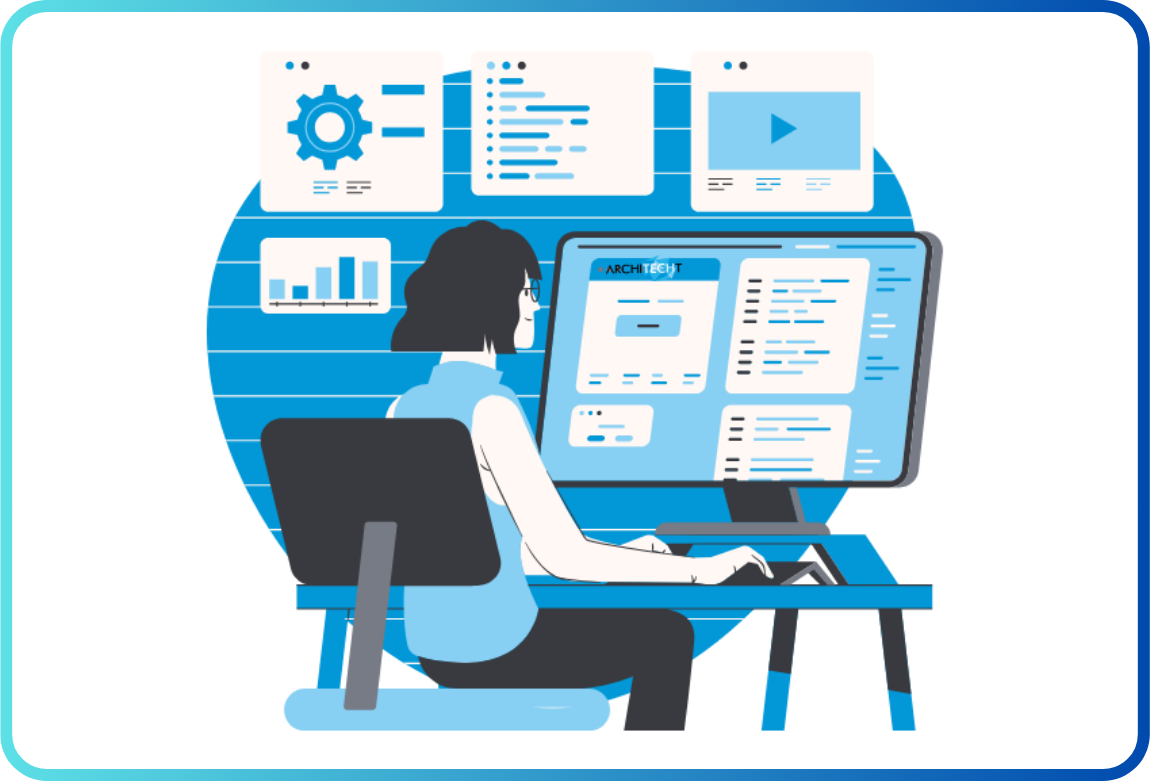
When navigating the web world, sometimes it can be time-consuming to move from one page to another, and that's where Single Page Applications (SPA) come into play. SPA offers a modern approach that allows users to access all content from just one page, making the web experience faster, smoother and more interactive.
Now let's examine the working logic of SPA step by step:
- Initial Load: When the user first enters SPA, the basic components of SPA, HTML, CSS and JavaScript files, are downloaded to the browser. These files allow the basic structure to be loaded into the browser.
- Client-Side Routing: SPA monitors the user's URL and determines which content will be displayed in the browser. When the URL changes, the page is not reloaded, only the relevant content is retrieved and displayed.
- API Requests and Data Retrieval: API Requests and Data Retrieval: As a result of user interactions, SPA makes API requests to get the necessary data from the server. These requests are usually realized with technologies such as AJAX or Fetch.
- Data Processing and Display: Data from the server usually comes in JSON format. This data is processed by the components within the SPA to create page content to be displayed to the user.
- Component-Based Structure: SPAs are formed by the combination of different components. Components represent different parts of the page (header, sidebar, content boxes, etc.).
- Content Update Without Page Reload: SPAs do not completely reload the page when the user clicks on a different link. Only the necessary data is retrieved and the content is dynamically updated. This allows users to navigate faster and have a smoother experience.
Figure – 1 Traditional Method and SPA working principle
Figure – 2: Comparison of Traditional Method and SPA
We have generally focused on the advantages of SPA, but what are its disadvantages? Let's examine this a little:
- SEO Challenges: Search engines may have difficulty indexing page content. In some SPAs, content that is not initially rendered server-side may be under-indexed by search engines.
- Memory Management: Since the application is constantly running, there may be memory leaks or performance issues. As page content grows, the amount of memory used in the browser may also increase.
- Initial Load Time: The initial load may require downloading and loading all the source files, which can result in a long wait at startup.
- Back Button Issues: The back button in the browser can sometimes have difficulty properly navigating past pages.
- Security: SPAs may require special attention to manage firewalls and authentication when exchanging data over RESTful APIs. They may impose increased responsibilities regarding data security.
- Device Compatibility: Some older browsers or devices may not support the required features of SPAs. This can lead to compatibility issues.
These disadvantages, despite the general advantages of SPAs, are factors that should be considered especially for certain scenarios or application requirements.
Let's try to better understand the SPA logic through an example application.
Let's consider the scenario of a restaurant reservation application:
ArchiNom Restaurant Reservation App Scenario:
A user wants to reserve a table for dinner using a restaurant reservation app called ArchiNom.
Traditional Website Scenario:
A user logs into the ArchiNom website and clicks on the “Book” section. A new page opens and all the reservation options are loaded. The user has to navigate through several pages to find a table available for the date and time they want. Each time, new pages are loaded and this process takes some time.
SPA Scenario:
A user visits the SPA version of ArchiNom. The homepage loads and all the reservation options are loaded once. The user uses the filters to search for a suitable table for the date and time they want. The page does not reload, only the tables that match the selected filters are listed instantly. The user can easily see the different times and options, no page refresh is required. When they finally find a suitable table, they follow the necessary steps to complete the reservation and the reservation process is completed quickly. This way, in the scenario where SPA is used, the user gets a faster and smoother experience because the page is not reloaded every time, only the necessary data is retrieved and the content is updated.
Let's create a simple SPA application together using Blazor:
First, let's create a model file for the data we need for the reservation.
ReservationModel.cs:
Then let's create our service file. This represents a service class where we can manage the business logic and operations required for reservation transactions. This file contains methods that include operations such as creating, updating, and deleting reservations. In addition, other operations required in the reservation process, such as database operations or communicating with external services, can be managed here.
ReservationService.cs:
Now we can start creating our pages. Let's have 3 pages.
1. Home
2. Reservation
3. Contact
First, let's create our landing page. General information about the application, images, etc. can be shared on this page.
Index.razor:
The ReservationForm.razor file creates the reservation form and receives input from the user. The HandleValidSubmit method is called when the form is validated and the reservation process is performed. The result is reported to the user.
ReservationForm.razor:
Let's create a page with contact information about the restaurant.
Contact.razor:
Finally, let's call these pages we created from a NavBar.
The NavMenu.razor file represents a component that manages the navigation of the Blazor application that supports the Single Page Application architecture. SPA works on a single HTML page when users navigate between pages in the application. This file supports the SPA features of the application by hosting components that include navigation, menu, and page redirections in the user interface.
NavMenu.razor:
When the links are clicked or the form is sent, the relevant content or reservation results are loaded into the main content section, providing a SPA-style reservation application experience.
The flexibility and performance advantages of SPA applications play an important role in modern web development processes. In this article, we have examined the working logic of SPA applications, their advantages and disadvantages in detail. We have also put these concepts into practice with a simple restaurant reservation application. While showing the areas of use and potential of single-page applications, we can foresee that the role of SPA will gradually increase in the construction of future web applications. With this change in technology, maximizing user experience and performance is now becoming more accessible. With new technological possibilities, the importance of SPA-based applications will continue to grow in future development processes.
REFERENCES
[1] - https://learningdaily.dev/blazor-web-development-building-single-page-applications-99d7fbf66c04
[2] - https://www.bloomreach.com/en/blog/2018/what-is-a-single-page-application
[3] - https://andrewly.medium.com/pros-and-cons-between-single-page-and-multi-page-apps-8f4b26acd9c9
[5] - https:// www.konstantinfo.com/blog/single-page-app-vs-multi-page-app/